There is no surprise that popups can be very powerful in enhancing conversion rate and boosting engagement when used the right way.
Now there are various ways you can set up a popup. But one effective type is the on-click popup, which appears only when a user clicks a specific element on a page.
There are two main ways to show a popup on button click:
1. Using JavaScript: JavaScript allows you to manually code an on-click popup that appears when a user clicks a button. You have full control over the design, behavior, and customization, but it requires coding knowledge and extra effort to manage.
2. Using A Plugin: A WordPress plugin like WowOptin lets you create on-click popups without coding. It comes with ready-to-use templates, advanced triggers, and analytics, making it a great choice for those who want an easy and powerful solution.
Each method has its own benefits. I will discuss both methods step-by-step in this article. You will also know details about on-click popup, examples, and their benefits in this blog. So, keep on reading!
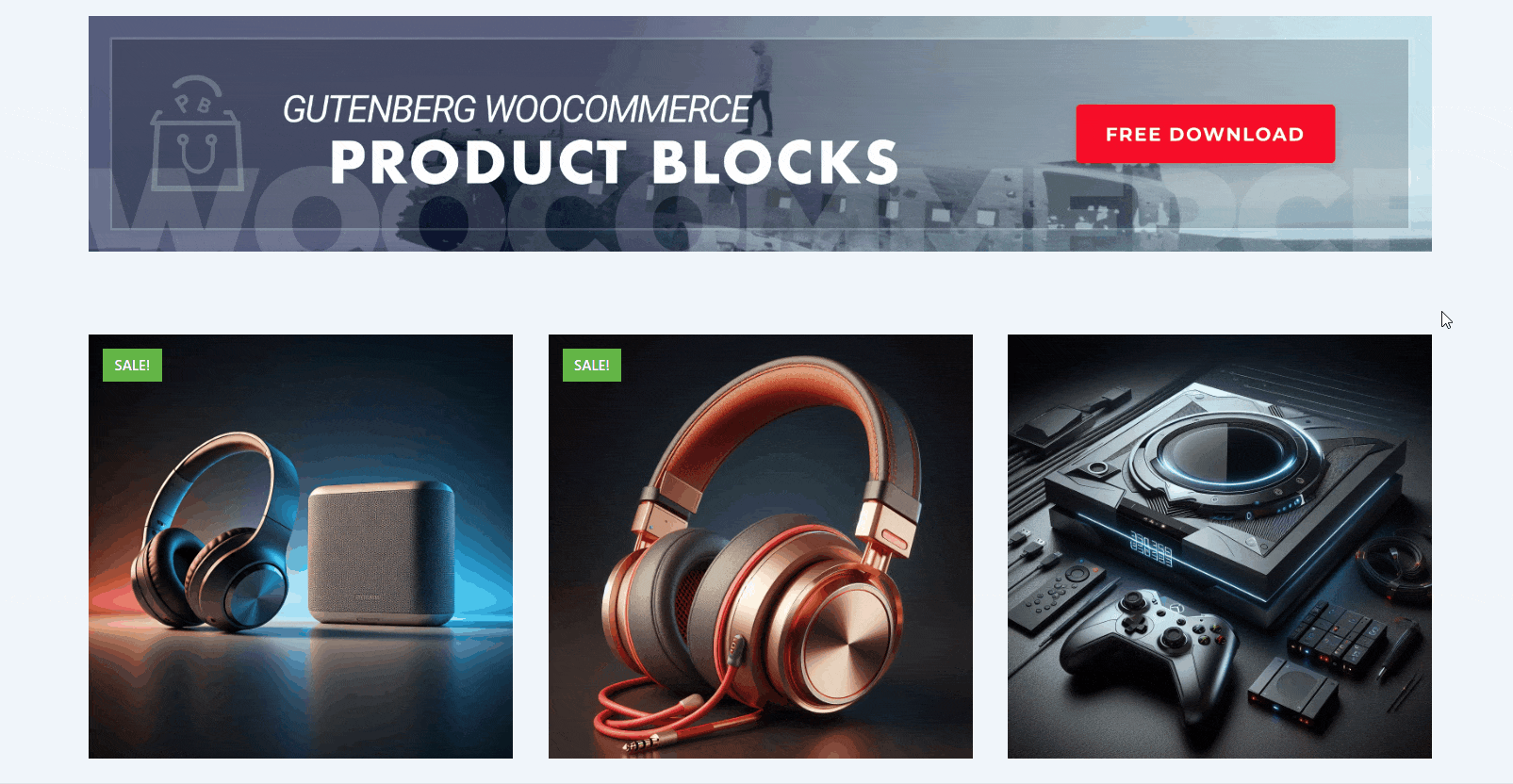
What is an On-Click Popup?
An on-click popup is a type of popup that only appears when a visitor clicks a button, link, or any other element on your page. Unlike annoying popups that appear immediately, this method ensures visitors see the popup only when they show interest.
How do you use it?
✅ Showing special offers after a user clicks “Get Discount”
✅ Displaying opt-in forms when a user clicks “Subscribe”
✅ Revealing additional product details in an eCommerce store
Examples of On-Click Popups
Still not sure how this works? Here are some real-world examples:
🔹 Discount Popups – A “Get 20% Off” button opens a popup with a coupon code.
🔹 Email Signups – A “Subscribe” button triggers an email signup form.
🔹 Product Information – A “More Details” button opens a popup with product specs.
🔹 Appointment Booking – Clicking “Book Now” brings up a booking form.
As you can see, you can serve different purposes by using a on-click popup. The possibilities are endless. This type of popup enhances user experience and boosts conversions without being intrusive.
But wait, there are more benefits. You will learn all about it in the next section:
Should You Implement an On-Click Popup on Your Site?
Okay, now you have a clear picture of what a click-triggered popup is. But what benefits do they bring for your site? Let’s find out.
They Improve User Experience
Popups can be a conversion booster, but can be annoying at the same time. But not true for on-click popup.
This type of popup does not appear out of nowhere, unlike other popups – it respects the user’s journey. Visitors only see them when they choose to engage.
They Lead to More Conversions
On-click popups depend on user interaction – meaning your offers or other messages in the popup will only be shown if the user is interested. So, there is a better chance the user will be converted.
For example, instead of showing offers directly, let your customers click on a “Get Offer” button. It will boost conversion as a result.
They Work for Any Type of Website
No matter what type of website you run. Let it be an eCommerce store, blog, SaaS platform, or service business, on-click popups can help you achieve your goals – without overwhelming visitors.
Method 1: Show Popup On Button Click Using Javascript
If you’re comfortable with basic HTML, CSS, and JavaScript, you can use this method. It gives you full control over how the popup will behave.
Step 1: Create the Button and Popup in HTML
First we need to set up the basic HTML file. For the click-triggered popup, we will require two elements:
- A button (#wowoptin-btn) that users will click.
- A popup container (#wowoptin-popup) that will be displayed when the button is clicked.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Show Popup on Button Click - WowOptin</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>Welcome to WowOptin</h1>
<p>Boost your conversions with powerful, targeted popups. Click below to see a sample popup in action.</p>
<!-- Button to Open Popup →
<button id="wowoptin-btn">Click to See Popup</button>
<!-- Popup Container →
<div id="wowoptin-popup">
<div class="popup-content">
<span class="close">×</span>
<h2>Try WowOptin Today!</h2>
<p>WowOptin helps you create high-converting popups with **no coding required**. Display personalized offers, collect leads, and boost your sales effortlessly.</p>
<a href="https://wowoptin.com/" target="_blank" class="wowoptin-link">Learn More</a>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Step 2: Add CSS for Basic Styling
Now, let’s add some CSS for the basic styling for the on-click popup.
/* Basic page styling */
body {
font-family: Arial, sans-serif;
text-align: center;
padding: 50px;
background-color: #f8f8f8;
}
/* Button styling */
#wowoptin-btn {
background-color: #0073e6;
color: white;
border: none;
padding: 12px 20px;
font-size: 16px;
border-radius: 6px;
cursor: pointer;
transition: 0.3s;
}
#wowoptin-btn:hover {
background-color: #005bb5;
}
/* Popup container - initially hidden */
#wowoptin-popup {
display: none;
position: fixed;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
background: white;
padding: 20px;
border-radius: 8px;
box-shadow: 0 5px 15px rgba(0, 0, 0, 0.3);
width: 320px;
text-align: center;
z-index: 1000;
}
/* Overlay Effect */
#wowoptin-popup::before {
content: "";
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background: rgba(0, 0, 0, 0.4);
z-index: -1;
}
/* Close button */
.close {
position: absolute;
right: 12px;
top: 8px;
font-size: 20px;
cursor: pointer;
color: #555;
}
.close:hover {
color: #000;
}
/* Learn More link */
.wowoptin-link {
text-decoration: none;
color: #0073e6;
font-weight: bold;
}
Step 3: Add JavaScript to Control the Popup
Now, we need JavaScript to make the popup appear when the button is clicked and disappear when the close button is clicked.
// Select the button and popup elements
const button = document.getElementById("wowoptin-btn");
const popup = document.getElementById("wowoptin-popup");
const closeBtn = document.querySelector(".close");
// Show popup when button is clicked
button.addEventListener("click", function() {
popup.style.display = "block";
});
// Hide popup when close button is clicked
closeBtn.addEventListener("click", function() {
popup.style.display = "none";
});
How This Works: (Step-by-Step Explanation)
1. We select the required elements from the HTML using JavaScript:
- #wowoptin-btn → The button that users click.
- #wowoptin-popup → The popup container that appears.
- .close → The close button inside the popup.
2. When the button is clicked (button.addEventListener(“click”, function() {})
- The popup’s display property is changed from none to block, making it visible.
3. When the close button is clicked (closeBtn.addEventListener(“click”, function() {})
- The popup’s display is set back to none, hiding it again.
Method 2: Show Popup On Button Click Using Plugin
If you want a simpler, more user-friendly method to create an on-click popup, you need to use a popup builder plugin. The one we recommend is WowOptin.
Why Use WowOptin?
✅ Canva-like editor – perfect for beginners.
✅ Advanced targeting – show popups to the right audience.
✅ Custom designs – fully customizable templates.
✅ One-time payment – no recurring fees.
Now, I will discuss how you can use WowOptin to trigger a popup on clicking a button in WordPress.
Step 1: Install & Activate WowOptin
To create a popup with WowOptin, you need to install and activate the plugin. The installation is similar to other plugins.
For more details, you can check out our documentation.
After installing the plugin, you will see a dashboard like this one.
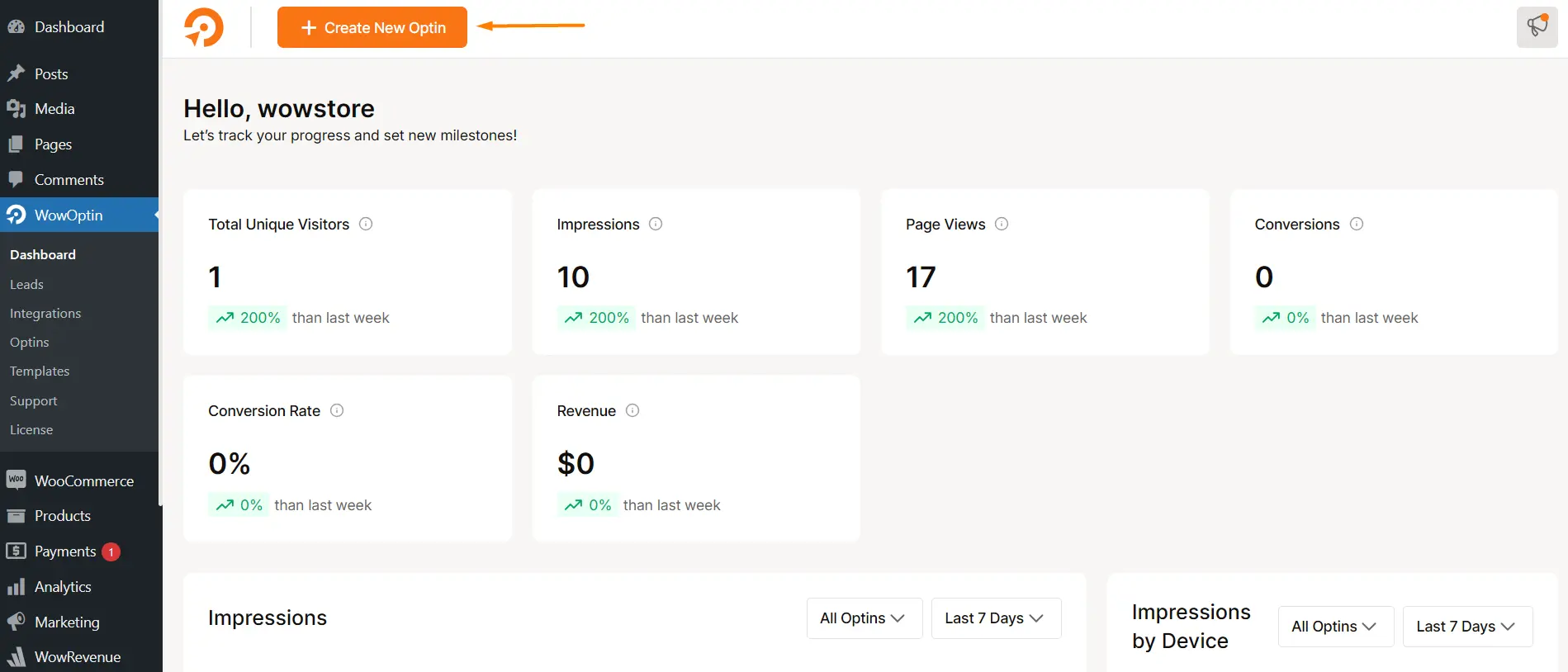
Step 2: Create A Popup with Preferred Elements
Next, create and design a popup that you want to display on your site and apply the on-click trigger.
After installing WowOptin, go to its dashboard and click on Create New Optin.
This will lead you to a new page where you can start with a premade popup template or create one from scratch. For now, I will use a premade one.
Now you will be in the popup editor view where you can add, remove, customize elements and set different conditions.
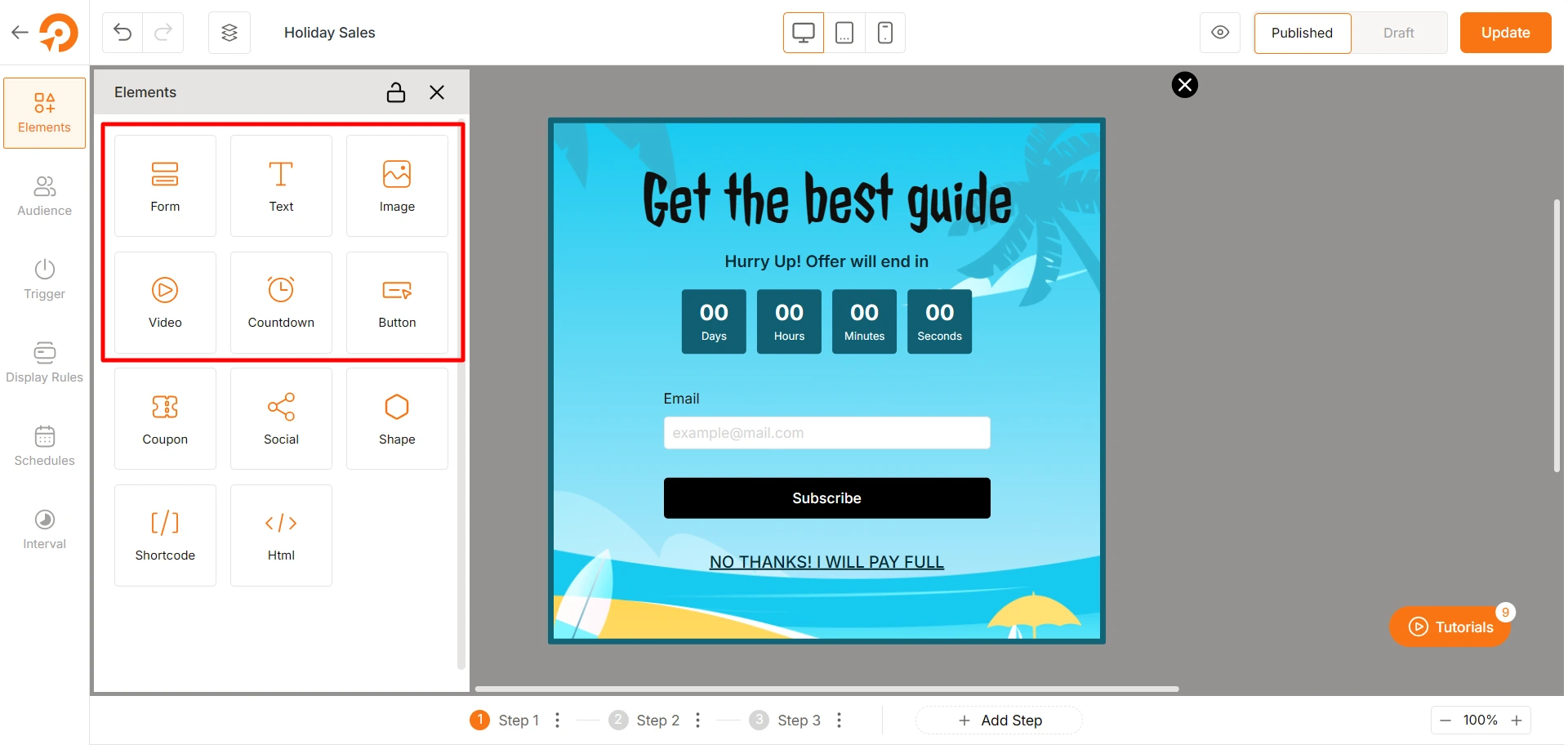
From the elements section, choose the ones that will make your popup look and function well.
If your goal is to open a popup form on button click, you should add a Form element from the list.
Step 3: Set up On-click Trigger
You gave the popup a stunning design, what now? The most important step – setting up the On-click trigger.
Move to the Trigger section and click on the Add New Trigger button. A dropdown list will show you available triggers offered by WowOptin.
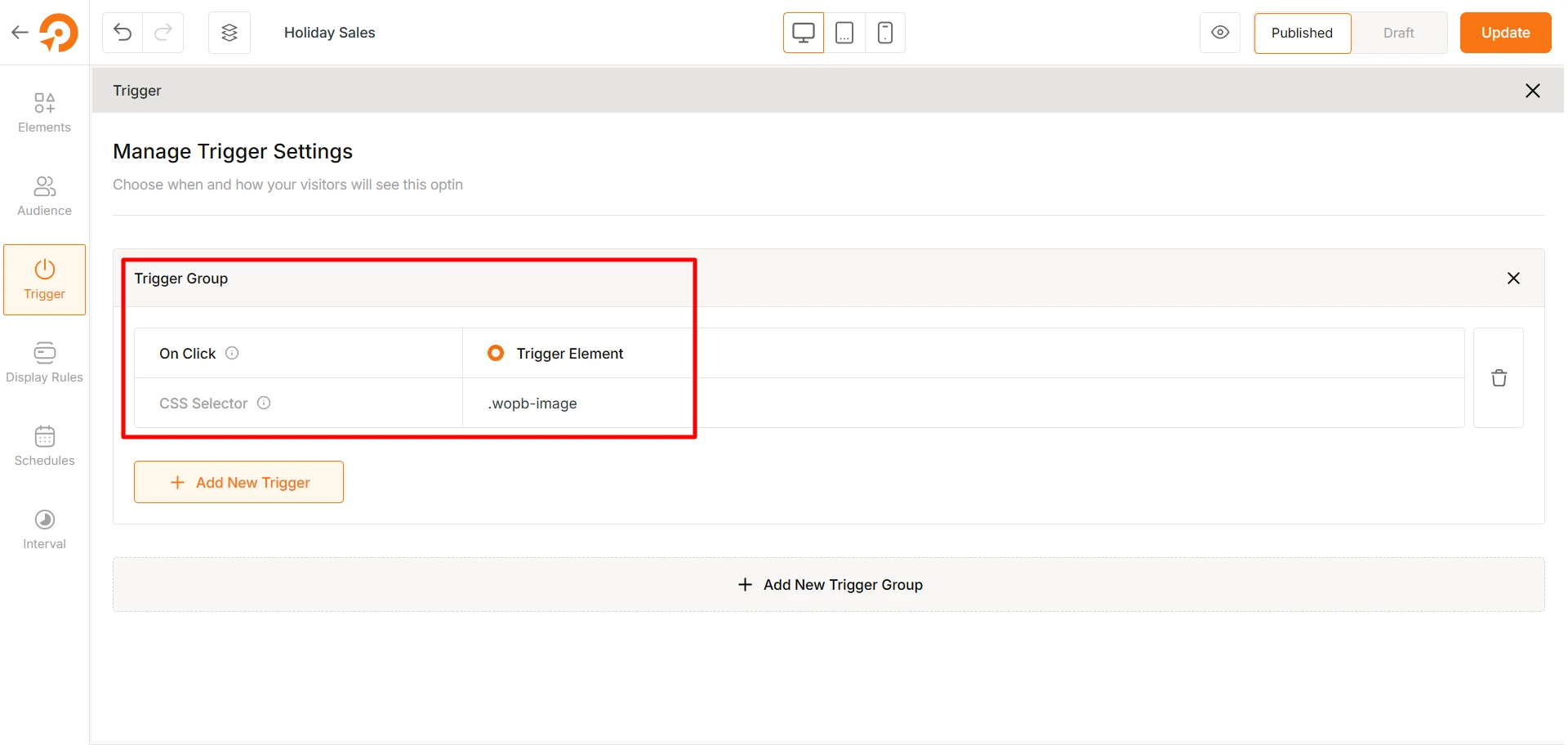
Choose the On-click option here. On the right side’s text field, put the CSS selector (class, id or tag) of the button for which the popup will be triggered.
To find the CSS class selector of a specific element on a page, right click on the element and choose Inspect. From here, you will see a class name. Copy the name and put a “.” in front of it and then paste it in the input field of Trigger Group from the above step.
Step 4: Additional Settings to Finalize the Popup
You have already set up the on-click triggered popup. But you should further apply other awesome features of WowOptin.
Choosing the Target Audience
From the Audience tab, you can choose the ideal audience for your on-click popup. If most of your customers come from a specific channel, or let’s say, use specific devices, you can set the options here.
Setting up Target Pages
Want to show the click-triggered popup on specific pages where the trigger element (such as the Subscribe button) is present? You can do that easily with WowOptin.
Simply move to the tab Display Rules. From here, you have the option to select the Entire Site (if the element is present in all pages), specific posts, posts from specific categories, cart page, product page and so on.
Scheduling the Popup
You can also schedule the popup to further control when it should be displayed. You can show it on specific days of the week, for special occasions, or on other custom schedules.
Frequently Asked Questions
Can I Open A Popup On Button Click Without Coding?
Yes, with WowOptin, you can easily create and display popups on button clicks without writing any code. WowOptin offers a no-code popup builder that lets you design and trigger popups using simple settings. Just create a popup, choose the “On Click” trigger, and link it to a button – and you are ready for success!
Can I Use Both Javascript And WowOptin Together to Create Popups?
Yes, you can use both in your website depending on your requirements. While WowOptin makes popup creation very easy, you can still create your own custom popup if you have coding knowledge.
However, using a dedicated plugin is recommended because it helps you manage the popups easily. For example, WowOptin lets you track the analytics of popups so that you can easily A/B test and optimize for best results.
How Can I Trigger A Popup on Page Load?
To trigger popup on page load, you need a popup builder plugin like WowOptin that features custom behavior. From its Interval settings, enable the option Every Page Load – to make the popup appear every time users refresh the page.
Do Popups Affect SEO?
It depends on how they are implemented. Intrusive popups that block the main content of the page and impact user experience can negatively affect SEO. However, on-click popups can be a good tactic because they only appear when users interact with the page.
How Do I Track Popup Conversions?
You can easily track your popups’ conversion using a plugin like WowOptin. These popup builder plugins include built-in analytics so you can easily see the impression, clicks, and other metrics for your popup..